本文章收录在黑鲸智能系统知识库
-黑鲸智能系统知识库成立于2021年,致力于建立一个完整的智能系统知识库体系。我们的工作:收集和整理世界范围内的学习资源,系统地建立一个内容全面、结构合理的知识库。
本文使用 Zhihu On VSCode 创作并发布Three.js 是一款运行在浏览器中的 3D 引擎,你可以用它创建各种三维场景,包括了摄影机、光影、材质等各种对象。你可以在它的主页上看到许多精彩的演示。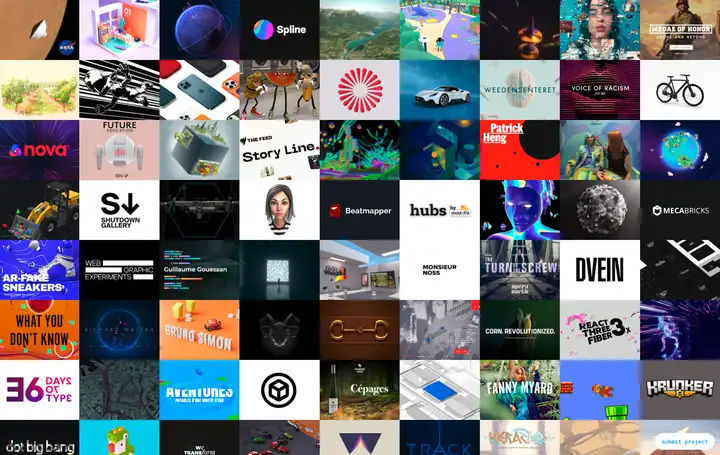
从现在起开始学习three.js作为以后开发AR项目的起步,每次看到别人做的炫酷的three.js应用都非常羡慕,大家如果对3D、AR等等概念有兴趣的话一定要了解一下,这篇文章作为我的three.js首篇笔记,简单介绍一下three.js的基本操作,已收录在黑鲸知识库里,欢迎大家关注后续的文章,也希望帮助不知道three.js的同学了解一下这个好玩的js库。如果大家感兴趣可以添加本站的交流微信群,一起讨论交流一下。
官方网站官方事例本站的交流微信群通过一个简单的例子来认识Three.js
为了真正能够让你的场景借助three.js来进行显示,我们需要以下几个对象:场景、相机和渲染器,这样我们就能透过摄像机渲染出场景, 下面是一个创建cube场景的小例子,你可以点击js查看源码及说明。See the Pen
Threejs练习1 by Kp (@uzizkp)
on CodePen.安装
NPM安装所有安装 three.js 的方式都依赖于 ES modules(参见 Eloquent JavaScript: ECMAScript Modules)。ES modules使你能够在最终项目中包含所需库的一小部分。
然后你就可以将它导入你的JS里代码了:
并非所有功能都可以通过 three 模块来直接访问(也称为“裸导入”)。three.js 中其它较为流行的部分 —— 如控制器(control)、加载器(loader)以及后期处理效果(post-processing effect) —— 必须从 examples/jsm 子目录下导入。
你可以从 Eloquent JavaScript: Installing with npm 来了解更多有关 npm 模块的信息。
通过CDN安装WebGL兼容性检查(WebGL compatibility check)
虽然这个问题现在已经变得越来不明显,但不可否定的是,某些设备以及浏览器直到现在仍然不支持WebGL。以下的方法可以帮助你检测当前用户所使用的环境是否支持WebGL,如果不支持,将会向用户提示一条信息。
请将https://github.com/mrdoob/three.js/blob/master/examples/jsm/WebGL.js引入到你的文件,并在尝试开始渲染之前先运行该文件。
画线
初步了解了three.js后,我们来尝试尝试一些简单的操作,比如画线
See the Pen
Three.js练习2 by Kp (@uzizkp)
on CodePen.载入3D模型
目前,3D模型的格式有成千上万种可供选择,但每一种格式都具有不同的目的、用途以及复杂性。 虽然 three.js已经提供了多种导入工具, 但是选择正确的文件格式以及工作流程将可以节省很多时间,以及避免遭受很多挫折。某些格式难以使用,或者实时体验效率低下,或者目前尚未得到完全支持。
如果有可能的话,推荐使用glTF(gl传输格式)。.GLB和.GLTF是这种格式的这两种不同版本, 都可以被很好地支持。由于glTF这种格式是专注于在程序运行时呈现三维物体的,所以它的传输效率非常高,且加载速度非常快。 功能方面则包括了网格、材质、纹理、皮肤、骨骼、变形目标、动画、灯光和摄像机。
公共领域的glTF文件可以在网上找到,例如 Sketchfab,或者很多工具包含了glTF的导出功能:
Blender by the Blender FoundationSubstance Painter by AllegorithmicModo by FoundryToolbag by MarmosetHoudini by SideFXCinema 4D by MAXONCOLLADA2GLTF by the Khronos GroupFBX2GLTF by FacebookOBJ2GLTF by Analytical Graphics Inc…and 还有更多加载
Three.js的examples文件夹里有少数加载器(如ObjectLoader)默认包含在three.js中例如使用loaders里的GLTFLoader来加载模型–其他加载器应单独添加到你的应用程序中。
一旦你引入了一个加载器,你就已经准备好为场景添加模型了。不同加载器之间可能具有不同的语法 —— 当使用其它格式的时候请参阅该格式加载器的示例以及文档。对于glTF,使用全局script的用法类似:
推荐使用glTF(gl传输格式)来对三维物体进行导入和导出,由于glTF这种格式专注于在程序运行时呈现三维物体,因此它的传输效率非常高,且加载速度非常快。
viewport相关的meta标签
这些标签用于在移动端浏览器上控制视口的大小和缩放(页面内容可能会以与可视区域不同的大小来呈现)。
MDN: Using the viewport meta tag
一些有用的链接(Useful links)
帮助论坛Three.js官方使用forum(官方论坛) 和 Stack Overflow来处理帮助请求。 如果你需要一些帮助,这才是你所要去的地方。请一定不要在GitHub上提issue来寻求帮助。
教程以及课程three.js入门
Three.js Fundamentals starting lessonBeginning with 3D WebGL by Rachel Smith.Animating scenes with WebGL and three.js更加广泛、高级的文章与教程
Discover three.jsThree.js FundamentalsCollection of tutorials by CJ Gammon.Glossy spheres in three.js.Interactive 3D Graphics – a free course on Udacity that teaches the fundamentals of 3D Graphics, and uses three.js as its coding tool.Aerotwist tutorials by Paul Lewis.Learning Three.js – a blog with articles dedicated to teaching three.jsThree.js Bookshelf– Looking for more resources about three.js or computer graphics in general? Check out the selection of literature recommended by the community.新闻与更新
Three.js on TwitterThree.js on redditWebGL on redditLearning WebGL Blog– The authoritive news source for WebGL.示例
three-seed – three.js starter project with ES6 and WebpackProfessor Stemkoskis Examples – a collection of beginner friendly examples built using three.js r60.Official three.js examples – these examples are maintained as part of the three.js repository, and always use the latest version of three.js.Official three.js dev branch examples– Same as the above, except these use the dev branch of three.js, and are used to check that everything is working as three.js being is developed.工具
physgl.org – JavaScript front-end with wrappers to three.js, to bring WebGL graphics to students learning physics and math.Whitestorm.js – Modular three.js framework with AmmoNext physics plugin.Three.js InspectorThreeNodes.js.comment-tagged-templates – VSCode extension syntax highlighting for tagged template strings, like: glsl.js.WebXR-emulator-extensionWebGL参考
webgl-reference-card.pdf – Reference of all WebGL and GLSL keywords, terminology, syntax and definitions.
暂无评论内容